Building a Drag & Drop kanban board with view transitions
Who said view transitions can't be used with React? We'll be adding animations to this kanban board in no-time. And on top of that, we explore the drag and drop API together.
... Almost ready!

Setting up the page layout
This video is part of Frontend.FYI PRO. A one-time purchase of €249 gives you access to all of our pro courses and paid tutorials. Including future releases.
The View Transitions API
Using the view transitions api is so easy, that I had to come up with some more interesting content to share in this video. That’s why we’ll be building a very basic Kanban board, where we’ll be adding drag and drop functionality, to then animate this with the view transitions API.
The HTML Drag and Drop API
The HTML Drag and Drop API allows us to make elements draggable, and make them droppable on other elements.
It all starts with the draggable
attribute, which we can add to any element. Setting the value to true
will make any element draggable like in the example below.
<div draggable="true">Drag me</div>
It might look like nothing special is happening when dragging the element. But this single line of code, enabled a lot of functionality for us.
Define a droppable target
Any element can be a droppable target. There’s two event handlers that can be listened to, to handle the drag and drop events.
These events are the onDragEnter
and onDragLeave
. These two events are fired when a draggable element respectively enters or leaves a droppable target. You can for example use this to highlight the droppable target when the draggable element enters it.
import { useState } from "react";import classNames from "classnames";
const AComponent = () => { const [isActive, setIsActive] = useState(false);
return ( <div> <div draggable="true">Drag me</div> <div className={classNames( "p-4 border-dashed border-2 border-gray-300", isActive && "bg-red-300", !isActive && "bg-transparent" )} onDragEnter={() => setIsActive(true)} onDragLeave={() => setIsActive(false)}> Drop here </div> </div> );}
Accept that drop
Finally, we need to do something if the user drops something on top of that target.
For that, the onDrop
event handler is what you’re looking for.
It does require some extra work though. Because by default, the browser will not allow you to drop anything on top of a droppable target.
To allow this, we need to prevent the default behavior of the onDragOver
event handler, like so:
import { useState } from "react";import classNames from "classnames";
const AComponent = () => { const [isActive, setIsActive] = useState(false);
return ( <div> <div draggable="true">Drag me</div> <div className={classNames( "p-4 border-dashed border-2 border-gray-300", isActive && "bg-red-300", !isActive && "bg-transparent" )} onDragEnter={() => setIsActive(true)} onDragLeave={() => setIsActive(false)} onDrop={() => { // Handle that drop. alert('Drop that beat!') }} // preventDefault can't be ommitted here. onDragOver={(ev) => ev.preventDefault()}> Drop here, and I'll tell you </div> </div> );}
That’s all there’s to it
With these few event handlers, you can create complicated drag and drop functionality, and build amazing experiences.
Now let’s add view transitions to the mix!
The view transitions API is a rather simple API, that allows you to animate between to states of you application. Or even two pages.
The API is based around two pieces: The viewTransitionName
property in CSS, and the JavaScript function document.startViewTransition
.
The viewTransitionName property
The browser animates any elements on the page, that have the same viewTransitionName
property between the two renders or pages. This means that you can animate elements that are not even on the same page, as long as they have the same viewTransitionName
property.
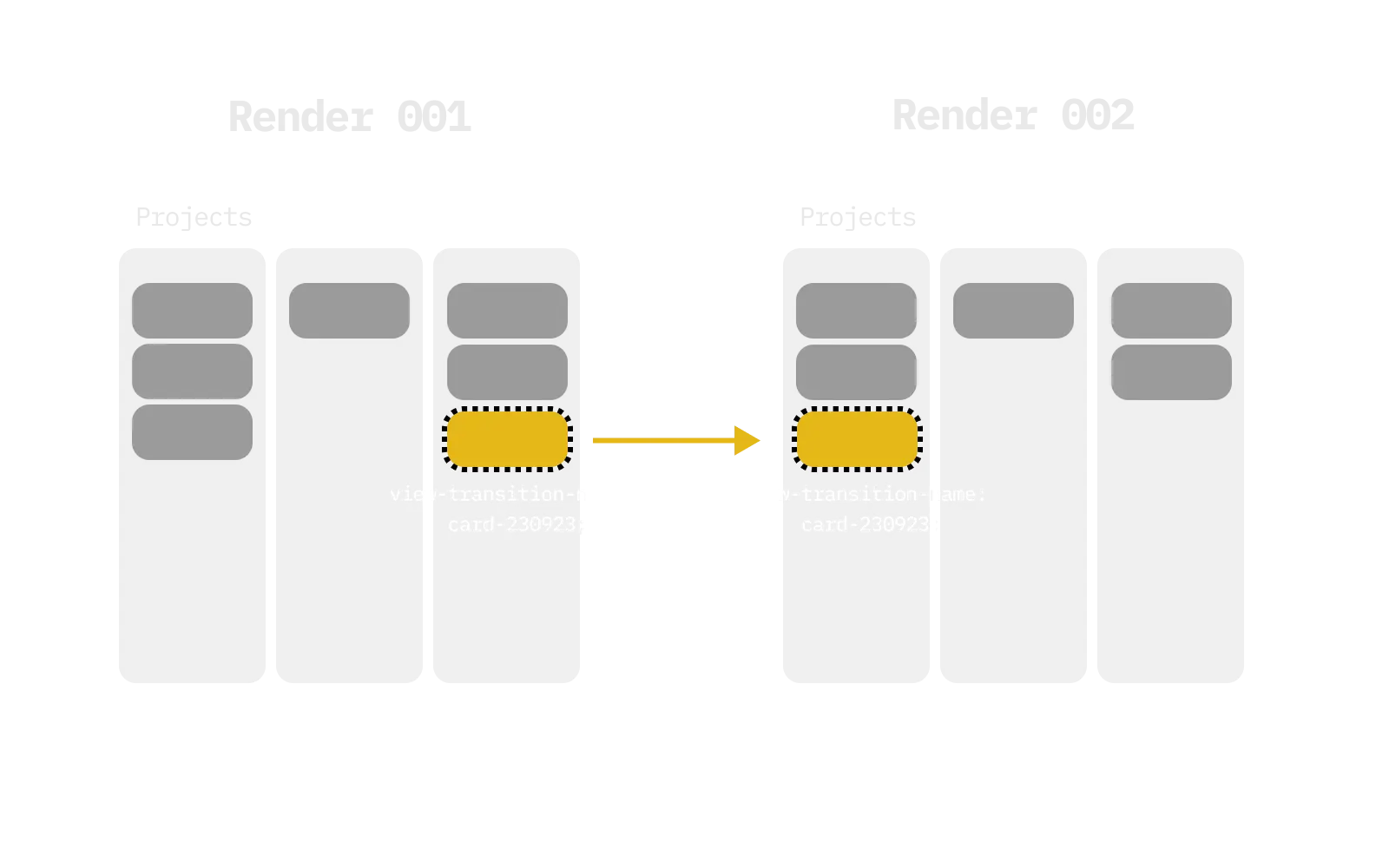
The document.startViewTransition function
This function allows you to inform the browser that an update in the DOM, which you’d like to be animated, is about to happen.
The browser expects you to run this function, and accepts a callback function as an argument. This callback function is the function that will update the DOM. The browser watch the changes made by this function, and will then animate the changes that are made in this callback function.
document.startViewTransition(() => { moveTheCards();});
Watch the video
Watch the video for a more in-depth explanation on how to use the view transitions API, and how to combine it with the drag and drop API.